VB.NET Filter() – Code Sample Syntax Example
Posted by asp.net videos on Sunday, February 20, 2011 · Leave a Comment
Premium (Not Free) Video Tutorials
Free Video Tutorials & Free Tools
Premium (Not Free) Video Tutorials
Free Video Tutorials & Free Tools
VB.NET Filter Example – Code Sample Syntax
Abstract: – Illustrates using VB.NET Filter Code Example.
*** 2. Filter Syntax ***
Purpose:
Returns a zero-based array containing a subset of a String array based on
specified filter criteria.
Syntax:
Filter (Source As String(), Match As String, Include As Boolean, _
Compare As CompareMethod) As String()
Parameters |
Description |
Source |
Required – one-dimensional string array to be searched
|
Match |
required – string to search for
|
Include |
optional – Boolean value indicating whether to return substrings that
include or exclude Match.
|
Compare |
optional – numeric value indicating compare method – CompareMethod.Binary
or CompareMethod.Text
|
Result Data Type |
Description |
array |
Returns a zero-based array containing a subset of a String array based on
specified filter criteria.
|
*** 3. Filter – Quick Example ***
|
Dim myStrings(3) As String
myStrings(0) = “This”
myStrings(1) = “is”
myStrings(2) = “my”
myStrings(3) = “string”
Dim FilteredStrings() As String
FilteredStrings = Filter(myStrings, “is”, True, CompareMethod.Text)
For Each myItem As String In FilteredStrings
Console.WriteLine(myItem) ‘ Returns [“This”, “Is”].
Next
|
*** 4. Filter – Full Example ***
Filter Example Output Screenshot
Step 1: Click Visual Basic to Cut-n-paste code into clsFilter.vb
Option Compare Text
Public Class clsFilter
Public Sub Main()
'****************************************************************************************
' Purpose: Returns a zero-based array containing a subset of a String array based on
' specified filter criteria.
'
' Syntax: Filter (Source As String(), Match As String, Include As Boolean, _
' Compare As CompareMethod) As String()
'
' Parameter1: Source - Required - one-dimensional string array to be searched
'
' Parameter2: Match - required - string to search for
'
' Parameter3: Include - optional - Boolean value indicating whether to return substrings that
' include or exclude Match.
'
' Parameter4: Compare - optional - numeric value indicating compare method - CompareMethod.Binary
' or CompareMethod.Text
'
' Result: array - Returns a zero-based array containing a subset of a String array based on
' specified filter criteria.
'
' Quick Example: Dim myStrings(3) As String
' myStrings(0) = "This"
' myStrings(1) = "is"
' myStrings(2) = "my"
' myStrings(3) = "string"
' Dim FilteredStrings() As String
' FilteredStrings = Filter(myStrings, "is", True, CompareMethod.Text)
' For Each myItem As String In FilteredStrings
' Console.WriteLine(myItem) ' Returns ["This", "Is"].
' Next
'
' *********************************************************
' Source - Required - one-dimensional string array to be searched
' Match - required - string to search for
' Include - optional - Boolean value indicating whether to return substrings that
' include or exclude Match.
' Compare - optional - numeric value indicating compare method - CompareMethod.Binary
' or CompareMethod.Text
'****************************************************************************************
Console.WriteLine("Example #1: Filter(Source, Match, Include,Compare) As String")
Dim myStrings(3) As String
myStrings(0) = "This"
myStrings(1) = "is"
myStrings(2) = "my"
myStrings(3) = "string"
Dim FilteredStrings() As String
FilteredStrings = Filter(myStrings, "is", True, CompareMethod.Text)
For Each myItem As String In FilteredStrings
Console.WriteLine(myItem) ' Returns ["This", "Is"].
Next
'write blank line to make output easier to read
Console.WriteLine()
'Prevent console from closing before you press enter
Console.ReadLine()
End Sub
End Class |
Option Compare Text
Public Class clsFilter
Public Sub Main()
'****************************************************************************************
' Purpose: Returns a zero-based array containing a subset of a String array based on
' specified filter criteria.
'
' Syntax: Filter (Source As String(), Match As String, Include As Boolean, _
' Compare As CompareMethod) As String()
'
' Parameter1: Source - Required - one-dimensional string array to be searched
'
' Parameter2: Match - required - string to search for
'
' Parameter3: Include - optional - Boolean value indicating whether to return substrings that
' include or exclude Match.
'
' Parameter4: Compare - optional - numeric value indicating compare method - CompareMethod.Binary
' or CompareMethod.Text
'
' Result: array - Returns a zero-based array containing a subset of a String array based on
' specified filter criteria.
'
' Quick Example: Dim myStrings(3) As String
' myStrings(0) = "This"
' myStrings(1) = "is"
' myStrings(2) = "my"
' myStrings(3) = "string"
' Dim FilteredStrings() As String
' FilteredStrings = Filter(myStrings, "is", True, CompareMethod.Text)
' For Each myItem As String In FilteredStrings
' Console.WriteLine(myItem) ' Returns ["This", "Is"].
' Next
'
' *********************************************************
' Source - Required - one-dimensional string array to be searched
' Match - required - string to search for
' Include - optional - Boolean value indicating whether to return substrings that
' include or exclude Match.
' Compare - optional - numeric value indicating compare method - CompareMethod.Binary
' or CompareMethod.Text
'****************************************************************************************
Console.WriteLine("Example #1: Filter(Source, Match, Include,Compare) As String")
Dim myStrings(3) As String
myStrings(0) = "This"
myStrings(1) = "is"
myStrings(2) = "my"
myStrings(3) = "string"
Dim FilteredStrings() As String
FilteredStrings = Filter(myStrings, "is", True, CompareMethod.Text)
For Each myItem As String In FilteredStrings
Console.WriteLine(myItem) ' Returns ["This", "Is"].
Next
'write blank line to make output easier to read
Console.WriteLine()
'Prevent console from closing before you press enter
Console.ReadLine()
End Sub
End Class
Step 2: Click Visual Basic to Cut-n-paste code into Module1.vb
Module Module1
Sub Main()
Dim myclsFilter As New clsFilter
myclsFilter.Main()
End Sub
End Module |
Module Module1
Sub Main()
Dim myclsFilter As New clsFilter
myclsFilter.Main()
End Sub
End Module
Prerequistes:
- Install Visual Basic (Express or Standard Edition)
- Install SQL Server Express
- Download Northwind and pubs Database
- Attach Northwind Database to Databases in Sql Express
- Attach pubs Database to Databases in Sql Express
Notes:
- Console Application is used to simplify things, but Windows Forms or Web Forms could also be used
- You can build a library of syntax examples by using same project over and over and just commenting out what you do not want to execute in Module1.vb
Instructions:
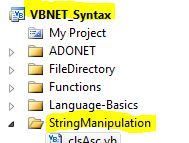
- Use Visual Basic 2010 Express or Standard Edition
- Create new project;
- Click File/New Project
- Select Console Application Template
- Select Visual Basic for Language
- name of project could be VBNET_Syntax.
- Add New folder named “StringManipulation”
- Right-click project name in solution explorer;
- add new folder;
- name of folder could be: StringManipulation
- Add Class Named clsFilter to StringManipulation folder
- Right-click StringManipulation folder;
- add new item;
- Select class
- Class name could be clsFilter
- Click on Visual Basic in code in step 1 above to copy code into clsFilter.vb
- Click on Visual Basic in code in step 2 above to copy code into Module1.vb
- Click green arrow or press F5 to run program
|